
roseau LOAD FLOW

roseau LOAD FLOW
A simple yet powerful power flow solver accessible through a Python API
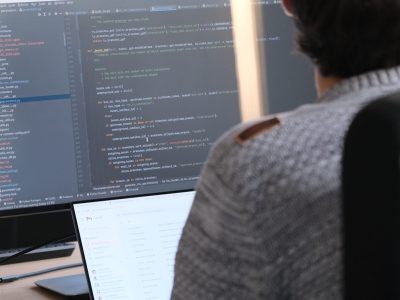
# Create two buses
source_bus = Bus(id="sb", phases="abcn")
load_bus = Bus(id="lb", phases="abcn")
# Define the reference of potentials to be the neutral of the source bus
ground = Ground(id="gnd")
pref = PotentialRef(id="pref", element=ground) # Fix the potential of the ground at 0 V
ground.connect(source_bus, phase="n")
# Create a LV source at the first bus
un = 400 / np.sqrt(3) # Volts (phase-to-neutral because the source is connected to the neutral)
source_voltages = Q_([un, un * np.exp(-2j * np.pi / 3), un * np.exp(2j * np.pi / 3)], "V")
vs = VoltageSource(id="vs", bus=source_bus, phases="abcn", voltages=source_voltages)
# Add a load at the second bus
load = PowerLoad(id="load", bus=load_bus, phases="abcn", powers=Q_([10 + 0j, 10 + 0j, 10 + 0j], "kVA"))
# Add a LV line between the source bus and the load bus
lp = LineParameters("lp", z_line=Q_((0.1 + 0.0j) * np.eye(4, dtype=complex), "ohm/km")) # R = 0.1 Ohm/km, X = 0
line = Line(id="line", bus1=source_bus, bus2=load_bus, phases="abcn", parameters=lp, length=Q_(2.0, "km"))
# Create the electrical network
en = ElectricalNetwork.from_element(source_bus)
# >>>
# Solve the load flow
activate_license(key="")
en.solve_load_flow()
# >>> 2 iterations
In need of a trustful load flow solver? We'll share ours!
Roseau Load Flow was originally developed for Roseau Technologies’ internal use, but good things are meant to be shared. That’s why we made a first public release of the solver in February 2023. Give it a try!
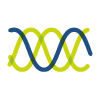
Static power network analysis
Standard load flow computation and other applications such as fault current calculation
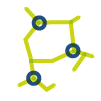
Full support of meshed networks, multiple phases and grid imbalance
Models of polyphase lines and transformers
-
Solves for unbalanced loading conditions and unbalanced faults
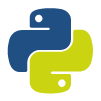
Accessible from a Python API
- Human-readable, object-oriented modeling of the grid
- Scripting for process automation
- Easy integration with other software
# Let's use a pq(u) control injecting reactive power
# before reducing active power
fp = FlexibleParameter.pq_u_production(
up_up=Q_(240, "V"),
up_max=250, # Volt by default
uq_min=Q_(200, "V"),
uq_down=Q_(210, "V"),
uq_up=Q_(235, "V"),
uq_max=Q_(240, "V"),
s_max=Q_(4, "kVA")
)
flexible_load = PowerLoad(
id="load",
bus=load_bus,
phases="abcn",
powers=Q_([-3.5, 0, 0], "kVA"),
flexible_params=[
fp,
FlexibleParameter.constant(),
FlexibleParameter.constant()
],
)
# Solve the load flow
en.solve_load_flow()
# >>> 2 iterations
# Get the results
abs(load_bus.res_voltages)
# >>> [242.72 232.62 233.68]
Fully generic modeling of loads and generators
- Library of standard loads (constant active/reactive loads, impedance loads…)
- Flexible generators can be finely modeled with P(U) and/or Q(U) curves and apparent power limits
- Generic design that makes it easy to add any new type of voltage-dependent loads/generators in the future
Simplicity and user-friendliness
- Synthetic, well-documented Python API
- Straightforward, transparent mathematical models: no transformation of the natural model, such as eliminating the neutral wire (Kron’s reduction) or changing the units to “p.u.”
- Support for physical units to avoid errors (e.g. confusing W and kW) and let everyone work with their preferred units (metric / imperial)
# Results per object
abs(load_bus.res_voltages).to("kV")
# >>> [0.22193 0.22193 0.22193]
abs(line.res_currents[0])
# >>> [45.06, 45.06, 45.06, 0. ]
# Global Results (data frame)
en.res_buses_voltages.transform([np.abs, np.angle]) # in V
# >>> voltage
# >>> absolute angle
# >>> bus_id phase
# >>> sb an 230.940108 6.671617e-37
# >>> bn 230.940108 -2.094395e+00
# >>> cn 230.940108 2.094395e+00
# >>> lb an 221.928183 2.599590e-22
# >>> bn 221.928183 -2.094395e+00
# >>> cn 221.928183 2.094395e+00
en.res_loads # in A, VA and V
# >>> current power potential
# >>> load_id phase
# >>> load a 45.06+0.00j 10000.00-0.00j 221.93-0.00j
# >>> b -22.53-39.02j 10000.00-0.00j -110.96-192.20j
# >>> c -22.53+39.02j 10000.00+0.00j -110.96+192.20j
# >>> n -0.00+0.00j -0.00+0.00j 0.00-0.00j
en.res_line_losses # in VA
# >>> series_losses shunt_losses total_losses
# >>> line_id phase
# >>> line a 406.07+0.00j 0.00+0.00j 406.07+0.00j
# >>> b 406.07+0.00j 0.00+0.00j 406.07+0.00j
# >>> c 406.07+0.00j 0.00+0.00j 406.07+0.00j
# >>> n 0.00+0.00j 0.00+0.00j 0.00+0.00j
Samples of grid data included
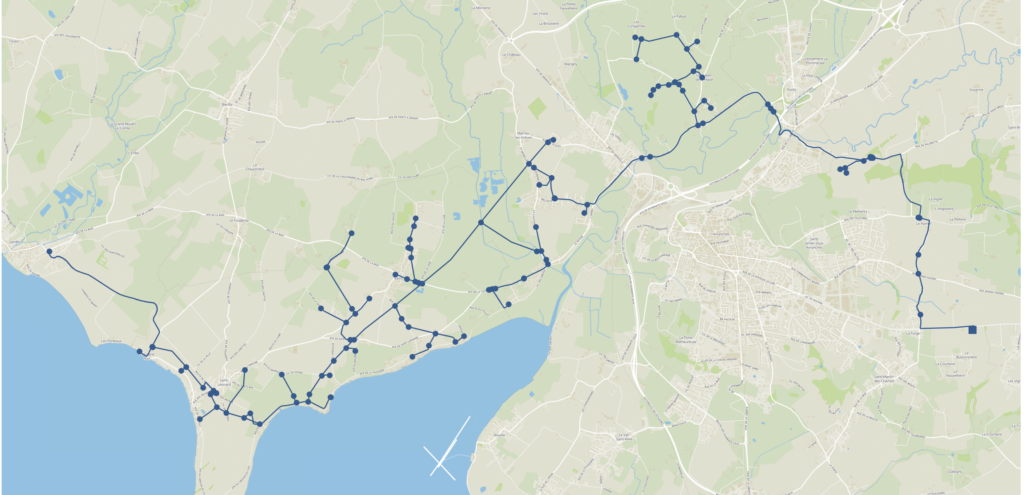
- Get started with ease thanks to the 20 Low Voltage and 20 Medium Voltage feeders included in Roseau Load Flow. Need to go further? We can provide you with the electrical model of the French distribution network, with tens of thousands of MV and LV feeders already modelled.
- Each network is given with its summer and winter load point.
- More info here!
Get your Roseau Load Flow licence
A free, public licence key is available for calculations on networks with up to ten nodes. Want to go further? Contact us to obtain your personal licence key.
Students and teachers: enter your academic email address below and you will receive your free licence key.
Installing and using Roseau Load Flow
Now that you’ve received your login details, let’s get started: